The fundamental React Hooks: A Comprehensive Guide to useState and useEffect
Welcome to the exciting world of React Hooks! If you're a budding developer or someone looking to level up your React skills, you've got to learn hooks.
In this article, we'll dive deep into two most used hooks in React: useState and useEffect.
React Hooks
These game-changing additions were introduced in React 16.8, revolutionizing the way we work with react. Developer could work without the need for class components with the help of hooks.
Hooks are JavaScript functions that provide a more concise way of writing reusable and maintainable code.
-
useState hook
-
useState hook enables functional components to have their own local state which was not possible before the release of react hooks.
-
It returns an array with state variable and a function to update that state respectively. We can also set a default value while defining the state.
-
Basic Syntax:
const [count, setCount] = useState(0); const handleChange = () => { // update the count value using setter method setCount(1); };
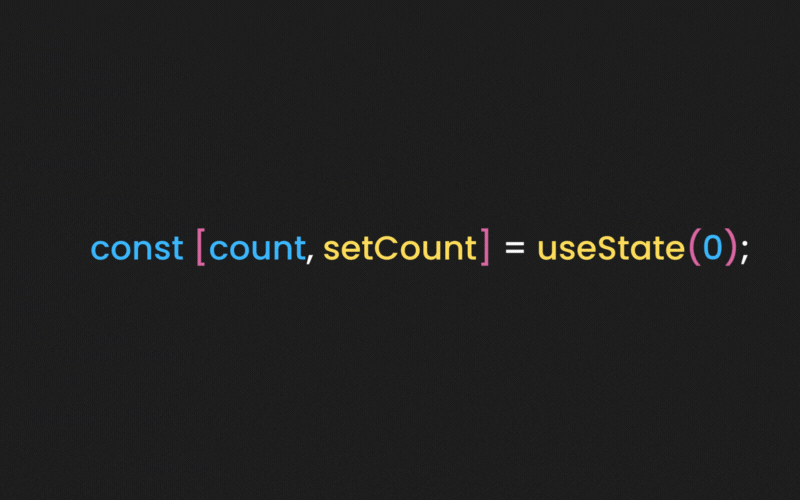
-
useState with Objects and Arrays:
const [user, setUser] = useState({ name: "", age: 0 }); const handleChange = () => { // update the state value using setter method setUser({ ...user, name: "john" }); };
The spread operator (...) is used to create a shallow copy of the current user object and update the relevant property.
In React, the useState hook is capable of storing values of various types, which includes fundamental data types like string, number, and Boolean, as well as more intricate ones like array, object, and function. In contrast, the state within a class component is constrained to object type only.
Essentially, any data that can be held within a JavaScript variable is viable for storage within a state managed through useState.
-
useEffect hook
The useEffect hook serves as a means for executing side effects within functional components. These side effects can include actions like data fetching, event listener setup, or DOM updates. It acts as a modern alternative to the traditional lifecycle methods found in class components, such as componentDidMount, componentDidUpdate, and componentWillUnmount.
The useEffect function accepts two parameters: firstly, a function that is executed after each render, and secondly, an array of dependency variables. When a dependency variable in this array is updated, the function is triggered, otherwise, it executes only after each render.
-
Basic Syntax:
useEffect(() => { /** * do someting everytime component renders */ }, []);
Example:
const [users, setUsers] = useState([]); useEffect(() => { fetch('https://jsonplaceholder.typicode.com/users') .then((response) => response.json()) .then((data) => setUsers(data)); }, []);
-
Syntax with dependencies:
useEffect(() => { /** * do someting everytime component renders and when the dependencies are updated */ }, [dep1, dep2, dep3]);
Example with dependency:
const [count, setCount] = useState(0); useEffect(() => { alert('Count is updated, new count is ', count) }, [count]); const handleChange = () => { // update the count value using setter method setCount(count++); };