How to conditionally render in React Functional Components | ReactJs Interview Questions
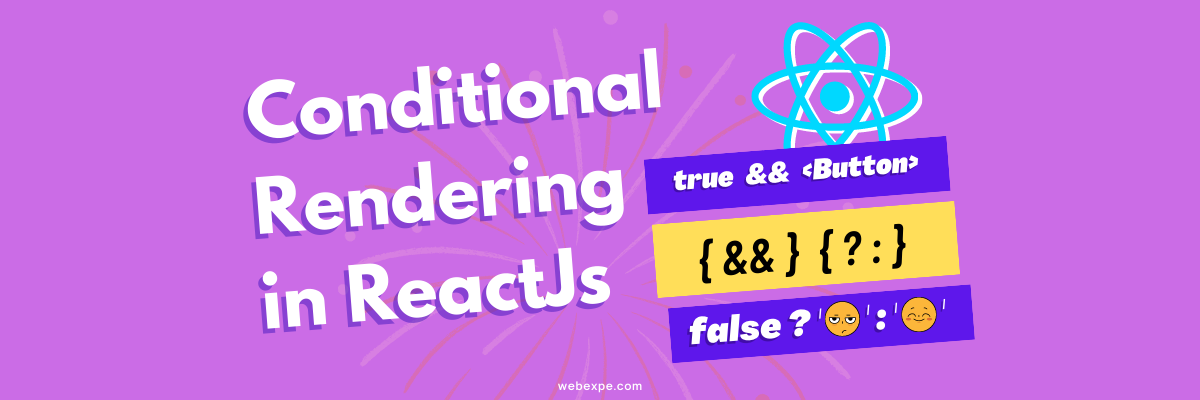
Conditional rendering refers to the ability of a component to display different content based on specific conditions.
It allows developers to control what elements are rendered and when they are rendered, depending on the state of the application or certain user interactions.
How to conditionally render in react
Let's look at the example below where we want to show a welcome message and a login button to the user depending on the props we get from parent component i.e. isLoggedIn. By default we have set it to false.
Conditional rendering is achieved by using JavaScript expressions inside the JSX (JavaScript XML) code. The expressions are evaluated at runtime, and the resulting content is rendered accordingly.
-
Using ternary operator
In the example below, we used a ternary operator (condition ? trueContent : falseContent) to conditionally render the welcome message.
import React from "react"; const Dashboard = ({ isLoggedIn = false }) => { return ( <div> { isLoggedIn ? <h1>Welcome, User!</h1> : <h1>Welcome, Guest!</h1> } </div> ); };
If isLoggedIn is true it will render Welcome, User! else it will render Welcome, Guest!.
-
Using inline logical AND operator
We also used a logical AND operator to render a button (condition && contentToBeShown)
import React from "react"; const Dashboard = ({ showLoginButton = true }) => { return ( <div> { showLoginButton && <button type="button"> Login </button> } </div> ); };
Here if showLoginButton is true it will render the Login button else it will render nothing.
Conclusion
Conditional rendering is a fundamental concept in React, as it enables dynamic and interactive user experiences.