A Complete Guide for Making API Calls in React Using Fetch and Axios with useEffect
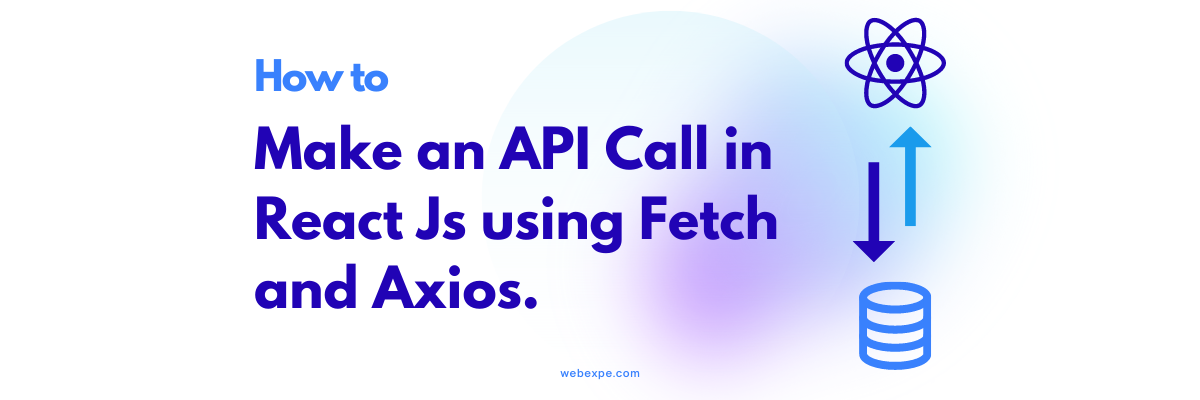
API requests are an important part of modern web applications. They enable the creation of dynamic, responsive web pages by allowing developers to access data from a variety of sources. In this article, we'll explore fetch, a method in JavaScript and axios, a library used to make API requests and learn how to use them with React to make api call, specifically by using the useEffect hook.
What is an API?
Before we dive into Fetch and Axios, let's first understand what is an API.
An API or Application Programming Interface, is a set of protocols, routines, and tools that allow different software applications to communicate with each other. APIs provide a standardized way for developers to access data and services from external sources, making it easier to build robust, integrated applications. Now that we know what an API is, let's get started with our topic!
Here's a step by step guide to make an API call:
Consider an example, where we want to fetch user list from the api on load of the component and display the list on the screen. We are going to use the JSONPlaceholder's users api. considering the json response looks like this, let's get started
[ { id: 1, name: "Leanne Graham", username: "Bret", email: "Sincere@april.biz", address: { street: "Kulas Light", suite: "Apt. 556", city: "Gwenborough", zipcode: "92998-3874", geo: { lat: "-37.3159", lng: "81.1496", }, }, phone: "1-770-736-8031 x56442", }, { id: 2, name: "Ervin Howell", username: "Antonette", email: "Shanna@melissa.tv", address: { street: "Victor Plains", suite: "Suite 879", city: "Wisokyburgh", zipcode: "90566-7771", geo: { lat: "-43.9509", lng: "-34.4618", }, }, phone: "010-692-6593 x09125", }, ];
-
Import useEffect
The first step is to import useEffect on top of your file.
import React, { useEffect } from "react";
-
Setup state variables
We will need to setup states for the following:
- user list that we fetch from the API
- error incase we run into some error while fetching the data
- a loading text to be shown on the screen while we fetch data
import React, { useState, useEffect } from "react"; const Example = () => { const [data, setData] = useState(null); const [error, setError] = useState(null); const [loading, setLoading] = useState(false); }; export default Example;
-
Write an useEffect
Now, we need to write the API call inside the useEffect hook.
The useEffect hook is a powerful hook that allows developers to handle side effects, such as API calls. A useEffect hook needs two parameter in our case, first, the callback function to make the API call and second, the empty dependency array.Passing an empty dependency array [] as a second argument indicates that the effect does not depend on any values from props or state and that the effect will only run on the initial render and not re-run on subsequent renders. That means the API call is only made once, when the component mounts.
-
Fetching data with fetch method
The fetch method is a built-in browser API in JavaScript is used to make a data request from a server. This means there is not need to install any library to use it. And Fetch only requires one parameter i.e. the api endpoint/url. Sounds pretty easy to use!
With this overview of fetch method, let's go ahead and in the callback method, first set loading to true and save the API data using setData function. Incase there is an error, we set the error using setError and accordingly show it on the screen to user.
import React, { useState, useEffect } from "react"; const UserList = () => { const [data, setData] = useState(null); const [error, setError] = useState(null); const [loading, setLoading] = useState(false); useEffect(() => { setLoading(true); fetch('https://fakeapi.com/user/'); // make the API call using fetch method .then(resp => resp.json()) .then(data => { setLoading(false); setData(data); }) .catch(error => { setLoading(false); setError(error); }); }, []); } export default UserList;
-
Making API call with Axios
Axios is a popular library that provides a more user-friendly interface for making API calls. Axios has a range of useful features, such as automatic data transformation, error handling and the ability to cancel requests. In the below example, we're using the Axios to make the same API call.
import React, { useState, useEffect } from 'react'; const UserList = () => { const [data, setData] = useState(null); const [error, setError] = useState(null); const [loading, setLoading] = useState(false); useEffect(() => { setLoading(true); axios.get('https://fakeapi.com/user/') .then(response => { setLoading(false); setData(response?.data); }); .catch(error => { setLoading(false); setError(error); }); }, []); } export default UserList;
-
-
Render the API data in JSX
Now that we have the list saved in the state, we display it using map method. If there is an error while fetching the data, we will show an error message. And during the data is fetched, we will show a loading message. Once the data is correctly retrieved, the user list would be displayed on the UI.
import React, { useState, useEffect } from "react"; const UserList = () => { const [data, setData] = useState(null); const [error, setError] = useState(null); const [loading, setLoading] = useState(false); useEffect(() => { setLoading(true); fetch("https://jsonplaceholder.typicode.com/users") .then((res) => res.json()) .then((data) => { setLoading(false); setData(data); }) .catch((error) => { setLoading(false); setError(error); }); }, []); return ( <div> {loading && <div>Loading...</div>} {error ? <div>Error: {error.message}</div> : null} {data?.length > 0 && data?.map((user) => ( <div> <div>Name: {user?.name}</div> <div>Address: {user?.address}</div> </div> ))} </div> ); }; export default UserList;
And that's it guys we have successfully made an API call on load of a component and displayed appropriate data.
Checkout the demo in the code sandbox below.
Conclusion
In this article, we've explored two powerful tools for making API calls in React: Fetch and Axios. We've also seen how to use the useEffect hook to make API calls.