How to create a star rating system with ReactJs, Emotion Js & React icons.
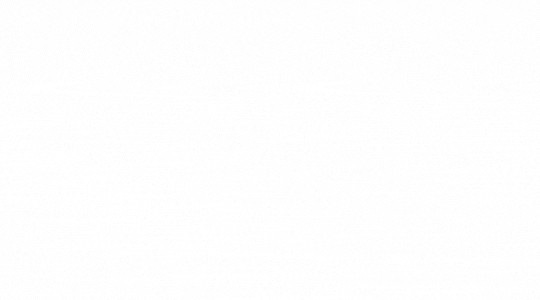
In this article, we'll walk through the steps to create a star rating system in React using the built-in React features such as state and event handlers. Let's dive right in!
Steps to create star rating sytem
-
Creating the Star rating component
Create a file with name StarRatingSystem.js and create a basic functional component named StarRatingSystem as shown below
const StarRating = () => {}; export default StarRating;
-
Required imports
To style our component, we'll use emotionJs and React-icons for the star icon. So we import AiFillStar icon from react-icons, css from emotion and useState from react as shown below.
import { css } from "@emotion/react"; import { useState } from "react"; import { AiFillStar } from "react-icons/ai";
-
Create state using useState hook
To know which star was clicked we need a state variable selectedStar and based on index/place of the star we will calculate how much rating the user has given which we will explore in more detail in the next step.
const StarRating = () => { const [selectedStar, setSelectedStar] = useState(); }; export default StarRating;
-
Logic for showing the colored stars
- The selectedStar state is basically going to contain the index of the star out of the 5 stars that has been clicked by the user. Initially set to a empty String
- In the jsx, we are going to check if the index of the individual star is less or equal to the selected star's index. If yes, we will change their color to yellow else it wilh have default grey color.
-
Creating and Styling the star rating system
Next, we'll create and add some styles to the stars to make it look like a star rating system. Create an array for 5 stars and, using map method we render 5 AiFillStar as shown below to avoid redundant code for the 5 stars.
As user is going to have to click on the stars, we will wrap each star with a button. Also, add some basic styling for the stars using emotionJS and pass that down to the AiFillStar using css prop
const text = css` font-size: 20px; text-align: center; `; const flex = css` margin-top: 50px; display: flex; justify-content: center; `; const star = css` margin-left: 10px; border: none; background: none; cursor: pointer; :hover { color: yellow; } `; const icon = css` font-size: 60px; `; const stars = [1, 2, 3, 4, 5]; const StarRating = () => { const [selectedStar, setSelectedStar] = useState(); return ( <> <div css={flex}> {stars.length > 0 && stars.map((starNum) => ( <button type="button" key={starNum} css={star}> <AiFillStar css={icon} /> </button> ))} </div> </> ); };
-
Add Onclick on the stars
When the user clicks a star we will capture the star's index and store it in the selectedStar state variable using setSelectedStar setter as shown below and compare each star's index with the selected star's index.
If individual star's index is less or equal to the selected star's index we will fill it with yellow color and if not, it will have color set to default grey color.
This can be done by sending a boolean value to the icon css method to display yellow or default grey color as shown in below code snippet below.
const icon = (isSelected) => css` color: ${isSelected ? "yellow" : "grey"}; font-size: 60px; `; const stars = [1, 2, 3, 4, 5]; const StarRating = () => { const [selectedStar, setSelectedStar] = useState(); return ( <> <div css={flex}> {stars.length > 0 && stars.map((starNum) => ( <button type="button" key={starNum} onClick={() => setSelectedStar(starNum)} css={star} > <AiFillStar css={icon( selectedStar === starNum || selectedStar > starNum )} /> </button> ))} </div> </> ); }; export default StarRating;
Time to run the code
As you can see we have 5 stars on the screen and when we click on the 4th star, we are able to display the correct rating.
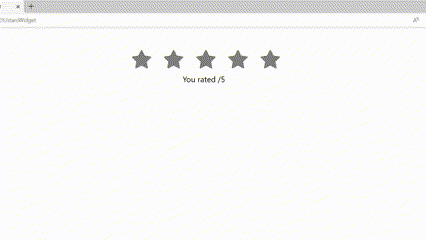
That's it! We've created a star rating system in React without using a third-party library.
Bonus!!😎 You can see this complete working code in the codesandbox below: