Class Components vs. Functional Components | React interview question.
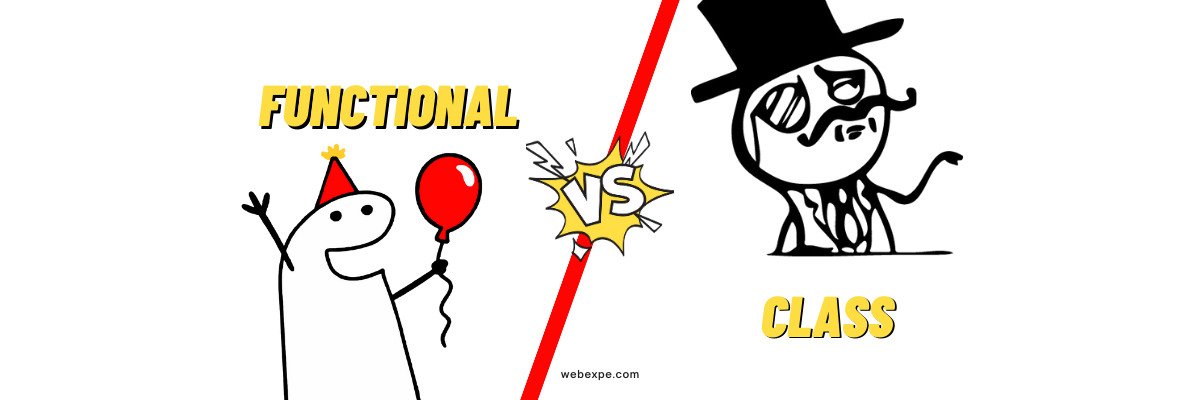
There are two main ways to create components in React: class components and functional components. In this article, we'll take a closer look at both types of components and explore the pros and cons of each. Let's get started.
Introduction to Class Components in React
Class components are the conventional method for creating components. They extend the React.Component base class and are built using a class function Object() . Before the release of React hooks, Class components were the best choice for complex components because they have access to lifecycle methods and state.
Here's a simple example of a class component in React
import React, { Component } from "react"; class Example extends Component { constructor(props) { super(props); this.state = { count: 0, }; } incrementCount = () => { this.setState({ count: this.state.count + 1, }); }; render() { return ( <div> <p>Count: {this.state.count}</p> <button onClick={this.incrementCount}>Increment</button> </div> ); } } export default Example;
Introduction to Functional Components in React
Functional components are stateless, simple JavaScript functions that can receive props as input and return React elements. They are easy to test, reuse and can't have lifecycle methods. But with Hooks, introduced in React 16.8, we could write state and complex state management logic in a functional component. This means that functional components can also handle complex logic, perform data fetching and updates, and manage state in a functional, reusable, and easy-to-understand way.
Here's a simple example of a functional component in React
import React, { useState, useEffect } from "react"; const Example = ({ title = "Counter" }) => { const [count, setCount] = useState(0); const [user, setUser] = useState({ name: "" }); useEffect(() => { const getDetails = async () => { const userDetails = await fetch("https://fakeapi/user"); // making an API call setUser(userDetails); }; getDetails(); }, []); return ( <div> <h1>{title}</h1> <h2>hello {user?.name}</h2> <p>Count: {count}</p> <button onClick={() => setCount(count + 1)}>Increment</button> </div> ); }; export default Example;
In the above example, we have used two most used hooks in a functional component.
- useState to manage the component state
- useEffect to make an API call on load or once the component has been mounted.
So, which one is the best? Which one should we use?
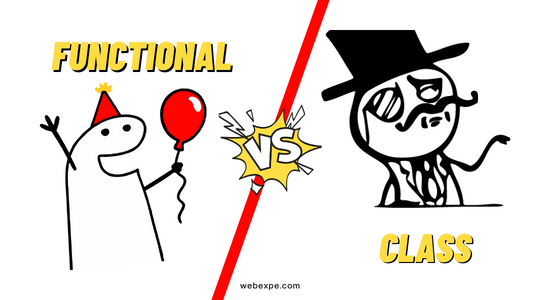
By now, you must have guessed it! Yes, Functional components are the best choice due to the advantages listed below.
Why to use Functional Components in React?
-
State management
Managing complex state and its side effects has become easier with introduction of React hooks in functional components
-
Concise
Functional components are more concise and require less code than class components.
-
Performance
Due to their simplicity and optimized logic for re-renders, functional components perform better than class components.
-
Easy to test
Functional components don't have implicit behaviour or need setup, therefore testing them is simple.