Understanding Controlled and Uncontrolled Components in ReactJS
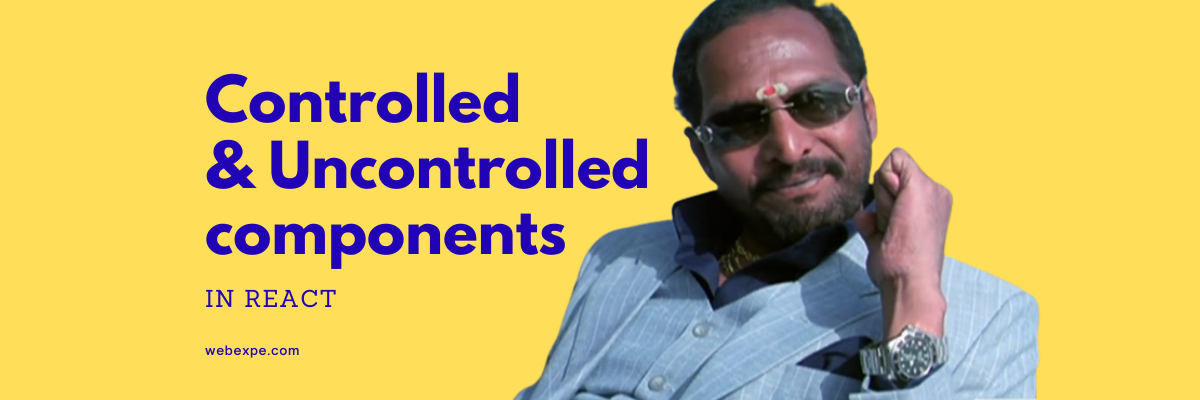
Controlled and uncontrolled components in ReactJS are two ways of managing the state of a component. Let's explore both of these in detail.
Controlled components
A controlled component is one where React manages the input's state and value. Any changes to the input are reflected in the state of the component, which ultimately updates the value of the input.
Example
In the below example, we have an input of type text and have used the useState hook to manage the state of the input. A value prop on the input element is set to the state value created by useState. The state is updated in the handleChange function, which is passed to the onChange prop of the input.
import React, { useState } from "react"; const ControlledInput = () => { const [value, setValue] = useState(""); const handleChange = (event) => { setValue(event.target.value); }; return <input type="text" value={value} onChange={handleChange} />; }; export default ControlledInput;
Here's a step-by-step explanation of what happens when the input value is updated:
- The user types something into the input field.
- The handleChange function is called and the new value of the input is passed as an argument to the function.
- The state is updated using setValue() with the new value.
- The component re-renders with the updated state and the value of the input is set to the updated state.
Uncontrolled components
An uncontrolled component is one where the DOM, rather than React, controls the input value. React has no control over how the input value is changed because the DOM does so directly.
Example
In the below example we have used useRef hook to create a reference to the input element. A reference is a way to access the DOM element directly. The input element has a ref prop that is set to the inputRef variable created using useRef hook. This allows us to access the value of the input directly through inputRef.current.value.
Here's a step-by-step explanation of what happens when the form is submitted:
- The user enters information and clicks the submit button.
- The handleSubmit function is called and preventDefault is used to prevent the default form submission behavior.
- the value of the input is logged to the console.
import React, { useRef } from "react"; const UncontrolledInput = () => { const inputRef = useRef(null); const handleSubmit = (event) => { event.preventDefault(); console.log(inputRef.current.value); }; return ( <form onSubmit={handleSubmit}> <input type="text" ref={inputRef} /> <button type="submit">Submit</button> </form> ); }; export default UncontrolledInput;
So, what is the conclusion? Which one should we use?
The answer is with "Uday Bhai". Yes, you read that correctly! 😂
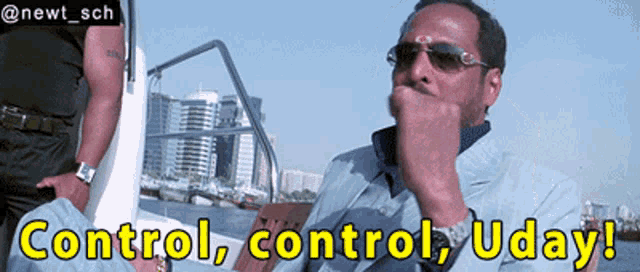
It is typically advised to use controlled components for more complex scenarios because uncontrolled components have some drawbacks. Here are a few reasons why using uncontrolled components is not a good idea.
-
Lack of Predictability
Uncontrolled components maintain their own internal state, which makes it harder to predict the state of the component at any given time. This can make debugging and maintaining the component more difficult. -
No Parent Control
With uncontrolled components, the parent component has no direct control over the state of the component. This can lead to unexpected behavior and make it difficult to ensure that the component is working as expected. -
Poor Performance
Uncontrolled components can lead to poor performance, especially in large applications, as the component must re-render every time the state changes. This can negatively impact the overall performance of the application. -
Security Issues
Because uncontrolled components have the capacity to alter their own states, they can potentially pose a security risk. Unexpected behaviour and possible security flaws may result from this. -
Complexity
Uncontrolled elements can quickly grow complex, especially when working with a lot of data. This may make it challenging to maintain and troubleshoot the component.
In conclusion, uncontrolled components have various drawbacks and it is advised to adopt controlled components for more complex scenarios because they give the component's state management a more predictable, secure, and maintainable way.